Banners for partners
With the banner functionality we provide a way to communicate to the customers offers and promotions.
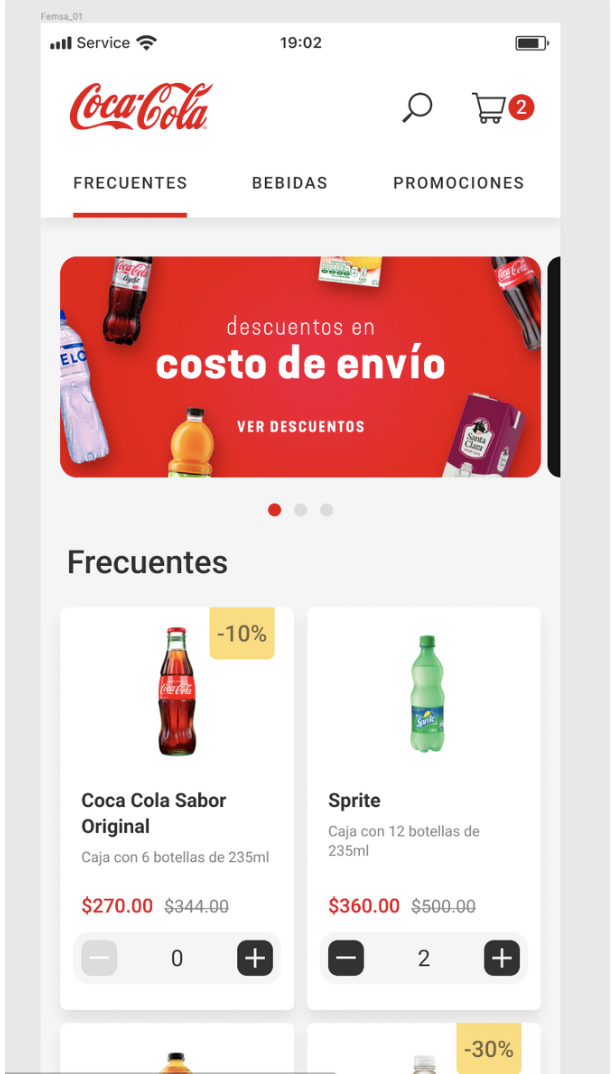
Admin API
In the Admin API we can found all the necessary functionalities to configure and create this banners.
Storefront configuration
To be able to use the banners functionality we should enable it in our storefront configuration.
{
"data": {
"configuration": {
"banner": {
"enabled": true
}
}
}
}
createBanner
This mutation is used to create a banner
mutation CreateBanner($storefrontName: String!, $data: BannerInput!) {
createBanner(storefrontName: $storefrontName, data: $data) {
id
imagesURL
customerType
reference
type
isActive
priority
startDate
expirationDate
}
}
Hereâs an example of the variables as JSON passed to the mutation:
{
"storefrontName": "test",
"data": {
"imagesURL": ["https://url/image"],
"customerType": "VIP",
"reference": "YAL100",
"type": "PRODUCT",
"isActive": true,
"priority": 1,
"startDate": "2022-08-01 11:00:00Z",
"expirationDate": "2022-09-01 11:00:00Z"
}
}
Name | Type | Required | Description |
---|---|---|---|
storefrontName | String | Yes | The storefront name where we want to create the banner |
imagesURL | Array String | Yes | This is an array of strings containing the paths to an image |
customerType | String | No | If the banner is for a specific type of customer. If not this field should be omitted and would be a default banner |
reference | String | No | If the banner is type PRODUCT this is required to contain the SKU of the product. For the others types is not a required field |
type | String | Yes | Only three possible values: PRODUCT , GENERAL , CATEGORY |
isActive | Boolean | No | If the banner is active for the user. The default value is true if the field is omitted |
priority | Int | No | The priority this banner has with the others, the default value if the field is omitted is 1 |
startDate | Date | Yes | The date when the banner will start to be available for the user |
expirationDate | Date | Yes | The date when the banner ends and it will not be showed to the user |
removeBanner
This mutation is used to remove a banner
mutation RemoveBanner($storefrontName: String!, $removeBannerId: ID!) {
removeBanner(storefrontName: $storefrontName, id: $removeBannerId) {
id
}
}
Hereâs an example of the variables as JSON passed to the mutation:
{
"storefrontName": "test",
"removeBannerId": "62fbad9f049e38dc5b2231da"
}
Name | Type | Required | Description |
---|---|---|---|
storefrontName | String | Yes | The storefront name where we want to remove the banner |
removeBannerId | String | Yes | The ID of the banner that we want to remove |
updateBanner
This mutation is used to update the fields of a banner
mutation UpdateBanner($storefrontName: String!, $updateBannerId: ID!, $data: BannerUpdate!) {
updateBanner(storefrontName: $storefrontName, id: $updateBannerId, data: $data) {
id
}
}
Hereâs an example of the variables as JSON passed to the mutation:
{
"storefrontName": "testdemo",
"updateBannerId": "62fa9cdfdab7c65501032322",
"data": {
"imagesURL": ["https://url/image"],
"customerType": "VIP",
"reference": "YAL100",
"type": "PRODUCT",
"isActive": true,
"priority": 1,
"startDate": "2022-08-01 11:00:00Z",
"expirationDate": "2022-09-01 11:00:00Z"
}
}
The descriptions of the fields available for update are the same that he ones listed here
banner
With this query we can retrieve the information of a specific banner by ID
query Banner($storefrontName: String!, $bannerId: ID!) {
banner(storefrontName: $storefrontName, id: $bannerId) {
id
imagesURL
customerType
reference
type
isActive
priority
startDate
expirationDate
}
}
Hereâs an example of the variables as JSON passed to the query:
{
"storefrontName": "testdemo",
"bannerId": "62fa9cdfdab7c65501032322"
}
Name | Type | Required | Description |
---|---|---|---|
storefrontName | String | Yes | The storefront name where we want to remove the banner |
bannerId | String | Yes | The ID of the banner that we want to retrieve |
banners
With this query we can retrieve a list of banners, this query can be paginated.
query Banners($storefrontName: String!, $pagination: PaginationInput) {
banners(storefrontName: $storefrontName, pagination: $pagination) {
id
imagesURL
customerType
reference
type
isActive
priority
startDate
expirationDate
}
}
Hereâs an example of the variables as JSON passed to the query:
{
"storefrontName": "testdemo",
"pagination": {
"pageNumber": 1,
"pageSize": 10
}
}
Name | Type | Required | Description |
---|---|---|---|
storefrontName | String | Yes | The storefront name where we want to remove the banner |
pageNumber | Number | No | The page we want to retrieve, if no value is specified a default of 1 will be used |
pageSize | Number | No | The number of items we want to retrieve for a page, if no value is specified a default of 10 will be used |
User API
In the User API we can found the functionality to obtain all the active banners configured that can be shown to a customer.
Get active banners
With this query we can retrieve the active banners for a customer. For a banner to be active the following rules should be satisfy:
- The storefront configuration should have the banners enabled
- The
isActive
property for the banner should betrue
- The todayâs date should be between the
startDate
and theexpirationDate
query Banners($storefrontName: String!, $sessionUid: ID!) {
banners(storefrontName: $storefrontName, sessionUid: $sessionUid) {
id
imagesURL
customerType
reference
type
isActive
priority
startDate
expirationDate
}
}
{
"storefrontName": "test",
"sessionUid": "62fa9cfb35743ccd20bd28df"
}
Session
The active banners can also be obtained using the query that retrieve the session by id
query Session($storefrontName: String!, $sessionId: ID!) {
session(storefrontName: $storefrontName, id: $sessionId) {
id
banners {
id
imagesURL
customerType
reference
type
isActive
priority
startDate
expirationDate
}
}
}
Hereâs an example of the variables as JSON passed to the query:
{
"storefrontName": "testdemo",
"sessionId": "62fa9cfb35743ccd20bd28df"
}
Updated about 1 year ago